ProblemIn a recent tip on Language Integrated Query (LINQ), you have described how it can be used as a query language extension to both VB.NET and C#. As XML has become a popular means to store data for ease of interoperability, how do we use LINQ to query XML data?
SolutionLanguage-Integrated Query for XML (LINQ to XML) allows XML data to be queried by using the standard query operators as well as tree-specific operators that provide XPath-like navigation through descendants, ancestors, and siblings. It simplifies working with XML data without having to resort to using additional language syntax like XPath or XQuery. You can use LINQ to XML to perform LINQ queries over XML that you retrieve from the file system, from a remote web service, or from an in-memory XML content. This tip will only focus on querying XML using LINQ from an XML file - theCustomers.xml file. You can download the XML file so you can follow along with the tip.
Create a simple LINQ project
We will start by following the steps outlined in the Introduction to LINQ tip. We will name our Visual Studio projectConsoleLINQtoXML and use the C# language. Add the following namespaces with the using directive. TheSystem.Xml.Linq namespace contains the classes for LINQ to XML
We will start by following the steps outlined in the Introduction to LINQ tip. We will name our Visual Studio projectConsoleLINQtoXML and use the C# language. Add the following namespaces with the using directive. TheSystem.Xml.Linq namespace contains the classes for LINQ to XML
//Include the LINQ to XML namespaces
using System.Xml; //namespace to deal with XML documents using System.Xml.Linq; //namespace to deal with LINQ to XML classes |
Next, we will add the Customers.xml file in our project. You can also opt to store the XML file in a directory in your file system but for simplicity's sake, we'll just include it in our project. Click on the Project menu and select Add Existing Item... from Visual Studio.
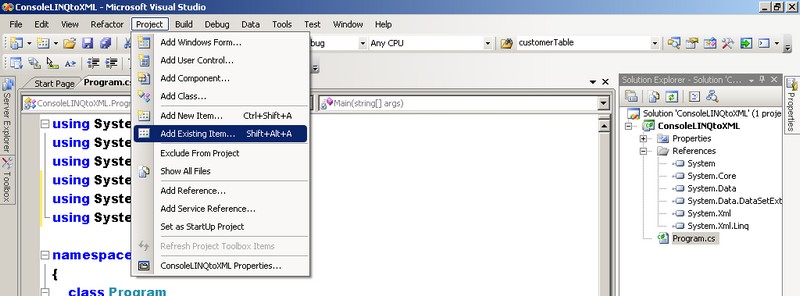
Select the Customers.xml file and click Add. The XML file should be added in your project.

Right-click on the Customers.xml file from the Solutions Explorer and select Properties. This should bring up the Properties window..
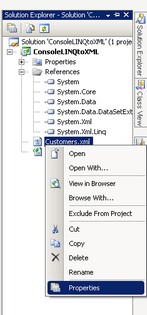
On the Properties window for the Customers.xml file, click the Copy to Output Directory option and select Copy always from the drop-down list
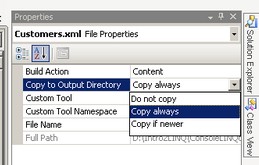
Now we are ready to write LINQ queries to read our XML file. Let's start writing some code inside the static voidMain(string[] args).
// Create the query
var custs = from c in XElement.Load("Customers.xml").Elements("Customers") select c ;
// Execute the query
foreach (var customer in custs) { Console.WriteLine(customer); } Console.ReadLine(); |
You will notice that there isn't much difference in the query from the Introduction to LINQ tip except for the fact that it now uses the XElement class which represents an XML element. The Load method of the XElement class simply loads the Customers.xml file into the XElement class. The Elements method returns a filtered collection of the child elements of this element - the Customers element -in the XML document. This just demonstrates how powerful LINQ is as you are using the same language query constructs on collections and now on XML documents. You can even implement sorting, filtering and grouping as you normally would in LINQ. You can insert a where clause in the query above to filter your results.
where c.Element("Country").Value == "Italy"
|
Your output will look like this when you run your project in Visual Studio. You can press F5 or click on Debug - Start Debugging in Visual Studio

Doing the same thing without LINQ is more complex as you still need to traverse the nodes explicitly to access the elements and their values. You can check out the Microsoft KB article that demonstrates how to do this in C# and compare the differences.
Next Steps
You have seen how easy it is to read XML documents with LINQ. You can use LINQ to read XML documents from the file system, from a remote web service, or from an in-memory XML content. We will look at using LINQ to XML to manipulate XML data in future tips.
- Learn more about LINQ for XML Data
No comments:
Post a Comment